Introduction:
Cross-Origin Resource Sharing, commonly known as CORS, is a security feature used to prevent multiple web browsers from requesting web pages to another domain that serves the original web page This security measure is important in case of a malicious attack in prevention and protection of sensitive user data. We’ll delve into CORS, its significance, and implement it effectively in a Node.js setting, exploring the concept thoroughly.
What is CORS?
CORS enables web pages to request restricted resources from domains different from their origin, promoting cross-origin resource sharing. It extends the same-origin policy (SOP), granting measured access to resources beyond a specific domain, enhancing flexibility. CORS doesn’t defend against CSRF but, if ineptly configured, exposes sites to potential cross-domain weaknesses.
The CORS workflow starts when a script loaded from one origin attempts to make a request to a resource on another origin. This workflow begins with the browser automatically making a preflight request to the external web server, which uses the HTTP method OPTIONS and has several HTTP headers that the external web server should validate to ensure that scripts are authorized to access the resource.
Why Would You Want to Implement CORS?
CORS, short for Cross-Origin Resource Sharing, addresses the same-origin policy, a security measure that stops a malicious script from accessing a different origin. Preventing cross-site request forgery (CSRF) issues relies on the significance of the same-origin policy, which thwarts the sending of fraudulent client requests from the victim’s browser to another application. CORS facilitates web app integration by permitting access to restricted resources across domains, useful for external API data retrieval and authorized third-party server resource access.
In summary, the primary reasons for implementing CORS include:
- Allowing web applications to interact with resources in different domains.
- Enabling the integration of applications and the use of third-party APIs.
- Allowing the pulling of data from external APIs or the access of server resources by authorized third parties.
How do CORS work?
CORS adds HTTP headers to client requests and server checks them before granting access to resources from a different origin.
When you make a cross-origin request, this is the request-response process:
- The browser adds an origin header to the request with information about the current origin’s protocol, host, and port.
- The server checks the current origin header and responds with the requested data and an Access-Control-Allow-Origin header.
- The browser sees the access control request headers and shares the returned data with the client applications.
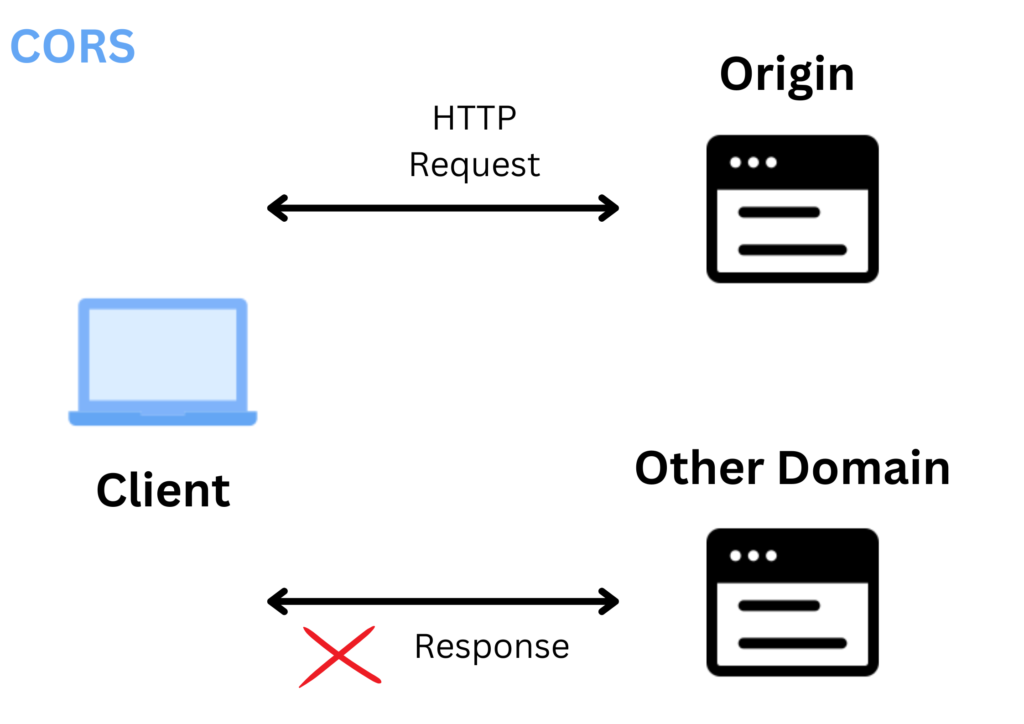
Why are we having this error?
The CORS error, often displayed as the “Same-Origin Policy” error, occurs when a web page running in one domain attempts to make a request to a resource hosted in a different domain, and the server hosting that resource doesn’t include the necessary HTTP headers to allow the request.
This error is a security measure designed to prevent malicious websites from making unauthorized requests to resources on other domains, which could potentially lead to data theft or other security vulnerabilities. The browser enforces the Same-Origin Policy by blocking the request if the necessary CORS headers are not present in the server’s response.
How can we solve it? and example with express CORS middleware
To solve these issues and enable cross-origin communication in your Node.js web application, you can use the Express.js middleware called ‘cors’.
Here’s how you can use it along with examples:
Install the package: You need to install the ‘cors’ package using npm or yarn.
npm install cors
Use the cors middleware in your Express app: Import and use it middleware in your Express application.
const express = require('express'); const cors = require('cors');
For all the requests
// Enable For all the Request const app = express(); app.use(cors()); // to all requests app.listen(3000, () => { console.log('Server started on port 3000') })
for specific request
const app = express() // for specific route app.get('/products/:id', cors(), function (req, res, next) { res.json({msg: 'For only product Route'}) }) app.listen(3000, function () { console.log('CORS-enabled web server listening on port 80') })
Customizing CORS Options:
The ‘cors‘ middleware can configure its behavior by providing options. For example, you can specify the allowed origins, the headers you can include in requests, and whether credentials (cookies) are allowed. Here are some examples:
const corsOptions = { origin: 'http://example.com', // replace with your allowed origin methods: 'GET,HEAD,PUT,PATCH,POST,DELETE', credentials: true, // enable credentials (cookies) optionsSuccessStatus: 204, // some legacy browsers (IE11, various SmartTVs) choke on 204 }; app.use(cors(corsOptions));
Conclusion:
Enabling CORS in a Node.js application is an important step when handling cross-origin requests. By understanding the importance of CORS and following the steps outlined above, you can ensure secure communication between your Node.js server and client-side applications. CORS policies may vary based on your specific application, so always check the official documentation for any other modification options.
Remember to be vigilant about best security practices and keep your applications safe from potential security threats. When used properly, CORS increases the overall security of your web applications, allowing for proper start-up communications.
Leave a Comment